Finally I added some LEDs on the 7-Segment display: a very bright set of 5 LEDs for the Weeling Flash indicator and a set of 5 standard red LEDs for the FUEL indication (if tank is empty, it should be ON).
Due to the uC IO max current limitation (I mean, the fact that the uC could not supply more then 2mA per IO), I have inserted a NPN transistor (BC337). Here the electrical circuit:
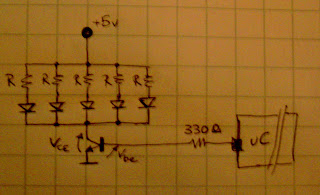
With very bright LEDs, the equation to calculate the series resistances is:
R = (5V - Vce - Vd) / Id
Where Vce is about 0.013V, Vd is the working LED voltage: Vd = 3V and Id is the working LED current: Id = 20mA (those walues are for very bright LEDs), therefore:
R = (5V - 0.013V - 3V) / 20mA = 100 Ohm.
While for normal LED (where Vd = 1.5V, Id = 10mA):
R = (5V - 0.013V - 1.5V) / 10mA = 360 Ohm
This is what the LEDs looks like after saldering on the DISPLAY board:
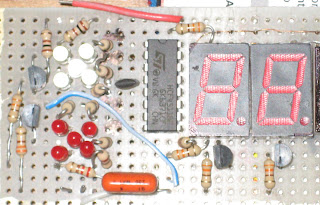
So, summarizing, the DISPLAY looks like:
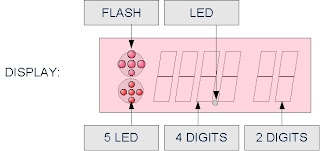
where are shown all the available display elements.
It is clear that the DISPLAY will show different informations depending on the weeling status, so for the
OFF status:
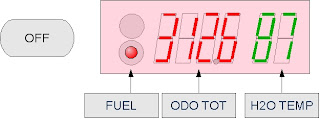
therefore each time the bike will stop for more then 3', the display will commute to shows engine water temperature, total distance and fuel status.
IDLE status:
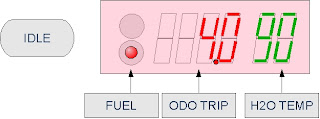
therefore each time the bike is in IDLE status (V < 5Hm/h), the display will commute to shows engine water temperature, ODO Trip and fuel status.
DRIVE status:
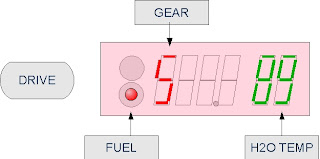
therefore each time the bike will drive, the display will commute to shows engine water temperature, gear and fuel status.
ACCELLERATING status:
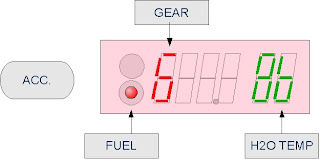
therefore each time the bike will accellerate, the display will commute to shows engine water temperature, gear and fuel status.
BOOST status:
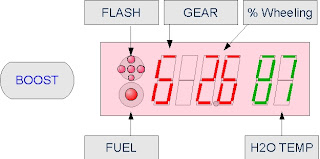
therefore each time the bike will accellerate very fast (with the risk of spinning), the display will commute to shows engine water temperature, gear, percentage of spinning and fuel status.
BRAKE status:
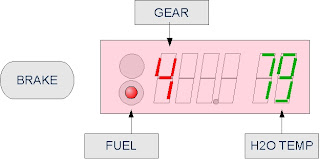
therefore each time the rider will brake, the display will commute to shows engine water temperature, gear and fuel status (while braking is very important to show the used gear to help the driver to avoid mistakes).
Software:
At the end, I decide to use a timer (TIMER 4) to refresh the display every 40Hz. This is to avoid flickering and a better software architecture. The 6 digits are store in:
unsigned char ucDisplayDigit[6]; // Display Buffer
every 25ms, the timer interrupt service will display one digit only, one by one. Therefore the display software is drawing one digit by one every 25msec (40Hz), the human eyes are integrating the light and the image happear as a whole (if refresh frequency is higher then 25Hz, it is also without flickering).
Here the interrupt service routine for Timer 4:
void GPT1_viTmr4(void) interrupt T4INT using RB_LEVEL9
{
// USER CODE BEGIN (Tmr4,2)
// USER CODE END
// USER CODE BEGIN (Tmr4,5)
if ( ucDisplayDigit[ucIdx] != 0xFF )
{
SelectDigit ( 0 ); // Deselect DIGIT
Display ( ucDisplayDigit[ucIdx] );
// DOT driver
P1L_P6 = ucDisplayDigit[ucIdx]>>4;
SelectDigit ( ucIdx );
}
ucIdx++;
if ( ucIdx > 5 ) ucIdx = 0;
// USER CODE END
} // End of function GPT1_viTmr4
As mentioned, the information to display are stored in the ucDisplayDigit character array. If a digit must not be displayed, then the respective value has to be 0xFF. If the relat DOT must be on, the forth digit must have the high nibble to 1 (i.e. 0x14 -> DOT is on, digit is 4). Here some further examples: